In this article, we will be solving HackerRank Day 7: Arrays problem, part of 30 Days of Code. Majorly, we will use Python3 and Java15 to solve the problem.
Objective:
Today we will learn about the Array Data Structure. Check out the Tutorial tab for learning materials and an instructional video.
Task:
Given an array, A, of N integers, print A‘s elements in reverse order as a single line of space-separated numbers.
Example
A = [1,2,3,4]
Print 4 3 2 1
. Each integer is separated by one space.
Input Format
The first line contains an integer, N (the size of our array).
The second line contains N space-separated integers that describe array A‘s elements.
Constraints
- 1 <= N <= 1000
- 2 <= A[i] <= 10000, where A[i] is the ith integer in the array.
Output Format
Print the elements of array A in reverse order as a single line of space-separated numbers.
Sample Input
4
1 4 3 2
Sample Output
2 3 4 1
You can find all the source code on my GitHub profile: https://github.com/uttammanani/HackerRank-30-Days-of-Code
Solution Day 7: Arrays in Python3
#!/bin/python3
import math
import os
import random
import re
import sys
if __name__ == '__main__':
n = int(input().strip())
arr = list(map(int, input().rstrip().split()))
print (*arr[::-1])
Solution Day 7: Arrays in Java15
import java.io.*;
import java.util.*;
public class Solution
{
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
int n = in.nextInt();
int[] arr = new int[n];
for(int i=0; i < n; i++) {
arr[i] = in.nextInt();
}
in.close();
for(int i=n-1; i >=0; i--) {
System.out.print(arr[i] + " ");
}
}
}
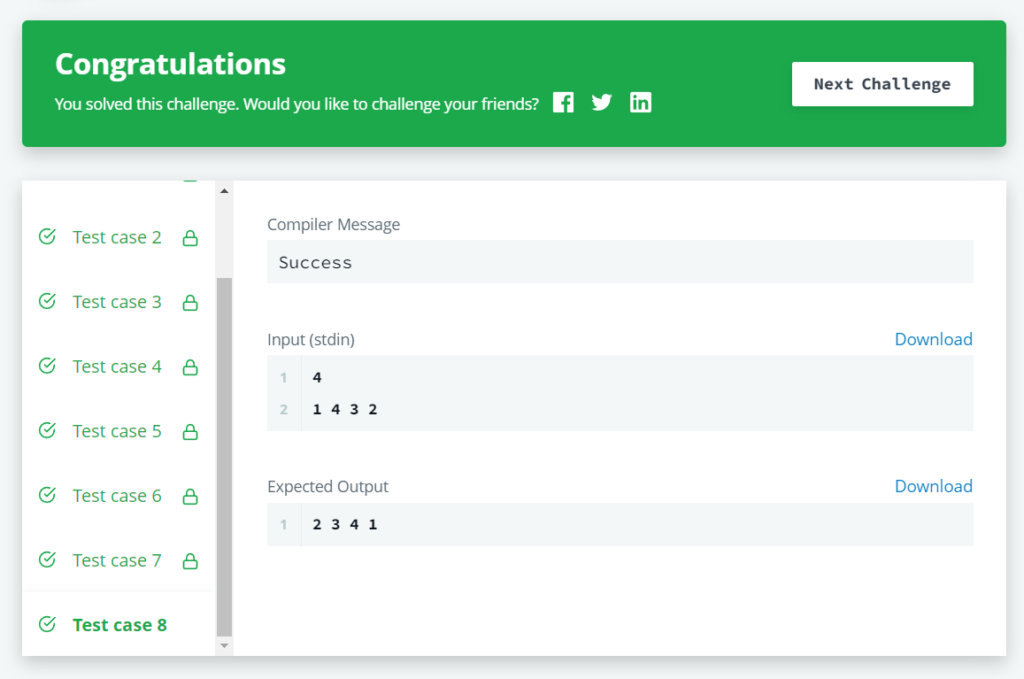
We hope that we bring some value to your life by posting our content, which might meet your expectations. You can always comment on the post to give feedback or reach out to us through email for sharing what you like to read on our blog.
Reach out to us using email: [email protected]
Find More Articles on Our Website: EGrasps
Check out our other posts on Medium.
You can reach out to us on WhatsApp.