In this article, we will be solving HackerRank Day 1: Data Types problem, part of 30 Days of Code. Majorly, we will use Python3 and Java15 to solve the problem.
Objective:
Today, we’re discussing data types. Check out the Tutorial tab for learning materials and an instructional video!
Task:
The variables i, d, and s are already declared and initialized. We must:
- Declare 3 variables, one of type int, one of type double, and one of type String.
- Read 3 lines of input from stdin and initialize your 3 variables.
- Use ‘+’ operator to perform the following operations:
- Print the sum of i plus your int varibale on a new line.
- Print the sum of d plus your double variable to a scale of one decimal place on a new line.
- Concatenate s with the string you read as input and print the result on a new line.
Input Format
- The first line contains an integer that you must sum with i.
- The second line contains a double that you must sum with d.
- The third line contains a string that you must concatenate with s.
Output Format
- Print the sum of both integers on the first line, the sum of both doubles (scaled to 1 decimal place) on the second line, and then the two concatenated strings on the third line.
Sample Input
12
4.0
is the best place to learn and practice coding!
Sample Output
16
8.0
HackerRank is the best place to learn and practice coding!
Explanation
- When we sum the integers 4 and 12, we get the integer 16.
- When we sum the floating-point numbers 4.0 and 4.0, we get 8.0.
- When we concatenate
HackerRank
withis the best place to learn and practice coding!
, we getHackerRank is the best place to learn and practice coding!
. - You will not pass this challenge if you attempt to assign the Sample Case values to your variables instead of following the instructions above and reading input from stdin.
You can find all the source code on my GitHub profile: https://github.com/uttammanani/HackerRank-30-Days-of-Code
Solution Day 1: Data Types in Python3
i = 4
d = 4.0
s = 'HackerRank '
int1 = int(input())
double1 = float(input())
string1 = input()
i += int1
d += double1
s += string1
print(i)
print(d)
print(s)
Solution Day 1: Data Types in Java15
import java.io.*;
import java.util.*;
public class Day1 {
public static void main(String[] args) {
/* Enter your code here. Read input from STDIN. Print output to STDOUT. Your class should be named Solution. */
int i = 4;
double d = 4.0;
String s = "HackerRank ";
Scanner scan = new Scanner(System.in);
// declaring user input variables
int i1;
double d1;
String s1;
// reading inputs
i1 = scan.nextInt();
d1 = scan.nextDouble();
scan.nextLine();
s1 = scan.nextLine();
// printing sum of int variable
System.out.println(i+i1);
// printing sum of double variable
System.out.println(d+d1);
// concatenating and print string
System.out.println(s.concat(s1));
scan.close();
}
}
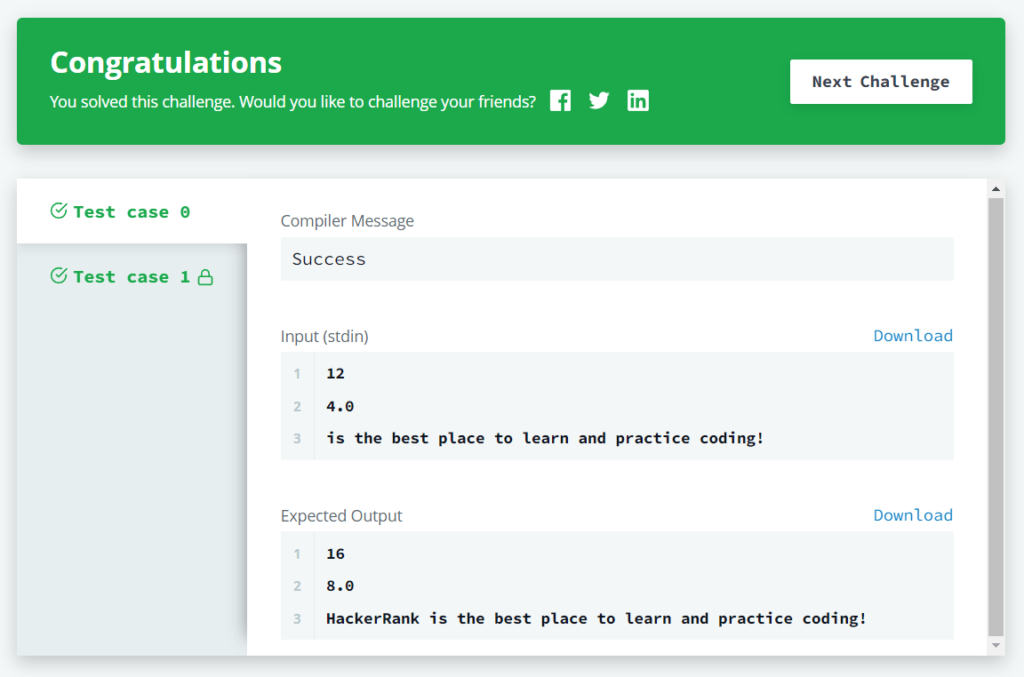
We hope that we bring some value to your life through posting our content, might content meet your expectations. You can always comment on the post to give feedback or reach out to us through email for sharing what you like to read on our blog.
Reach out to us using email: [email protected]
Find More Articles on Our Website: EGrasps
Check out our other posts on Medium.
You can reach out to us on WhatsApp.