In this article, we will be solving HackerRank Day 2: Operators problem, part of 30 Days of Code. Majorly, we will use Python3 and Java15 to solve the problem.
Objective
In this challenge, you will work with arithmetic operators. Check out the Tutorial tab for learning materials and an instructional video.
Task:
Given the meal price (base cost of a meal), tip percent (the percentage of the meal price being added as a tip), and tax percent (the percentage of the meal price being added as tax) for a meal, find and print the meal’s total cost. Round the result to the nearest integer.
Example:
- mealCost = 100
- tipPercent = 15
- taxPercent = 8
A tip of 15% * 100 = 15, and the taxes are 8% * 100 = 8. Print the value 123 and return from the function.
Function Description
Complete the solve function in the editor of HackerRank.
Function solve has the following parameters:
- int meal_cost: the cost of food before tip and tax
- int tip_percent: the tip percentage
- int tax_percent: the tax percentage
Returns The function returns nothing. Print the calculated value, rounded to the nearest integer.
Note: Be sure to use precise values for your calculations, or you may end up with an incorrectly rounded result.
Input Format
There are 3 lines of numeric input:
- The first line has a double, mealCost (the cost of the meal before tax and tip).
- The second line has an integer, tipPercent (the percentage of mealCost being added as tip).
- The third line has an integer, taxPercent (the percentage of mealCost being added as tax).
Sample Input
12.00
20
8
Sample Output
15
Explanation
Given:
meal_cost = 12, tip_percent = 20, tax_percent = 8
Calculations:
- tip = 12 and ((12/100) * 20) = 2.4
- tax = 8 and ((8/100) * 20) = 0.96
- total_cost = meal_cost + tip + tax = 12 + 2.4 + 0.96 = 15.36
- round(total_cost) = 15
- We round total_cost to the nearest integer and print the result, 15.
You can find all the source code on my GitHub profile: https://github.com/uttammanani/HackerRank-30-Days-of-Code
Solution Day 2: Operators in Python3
#!/bin/python3
import math
import os
import random
import re
import sys
#
# Complete the 'solve' function below.
#
# The function accepts following parameters:
# 1. DOUBLE meal_cost
# 2. INTEGER tip_percent
# 3. INTEGER tax_percent
#
def solve(meal_cost, tip_percent, tax_percent):
# Write your code here
tip = (tip_percent * meal_cost) / 100
tax = (tax_percent * meal_cost) / 100
total_cost = round(meal_cost + tip + tax)
print(total_cost)
if __name__ == '__main__':
meal_cost = float(input().strip())
tip_percent = int(input().strip())
tax_percent = int(input().strip())
solve(meal_cost, tip_percent, tax_percent)
Solution Day 2: Operators in Java15
import java.io.*;
import java.math.*;
import java.security.*;
import java.text.*;
import java.util.*;
import java.util.concurrent.*;
import java.util.function.*;
import java.util.regex.*;
import java.util.stream.*;
import static java.util.stream.Collectors.joining;
import static java.util.stream.Collectors.toList;
class Result {
/*
* Complete the 'solve' function below.
*
* The function accepts following parameters:
* 1. DOUBLE meal_cost
* 2. INTEGER tip_percent
* 3. INTEGER tax_percent
*/
public static void solve(double meal_cost, int tip_percent, int tax_percent) {
// Write your code here
double tip=meal_cost*tip_percent/100;
double tax=meal_cost*tax_percent/100;
int totalCost=(int)Math.round(meal_cost+tip+tax);
System.out.print(totalCost);
}
}
public class Solution {
public static void main(String[] args) throws IOException {
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(System.in));
double meal_cost = Double.parseDouble(bufferedReader.readLine().trim());
int tip_percent = Integer.parseInt(bufferedReader.readLine().trim());
int tax_percent = Integer.parseInt(bufferedReader.readLine().trim());
Result.solve(meal_cost, tip_percent, tax_percent);
bufferedReader.close();
}
}
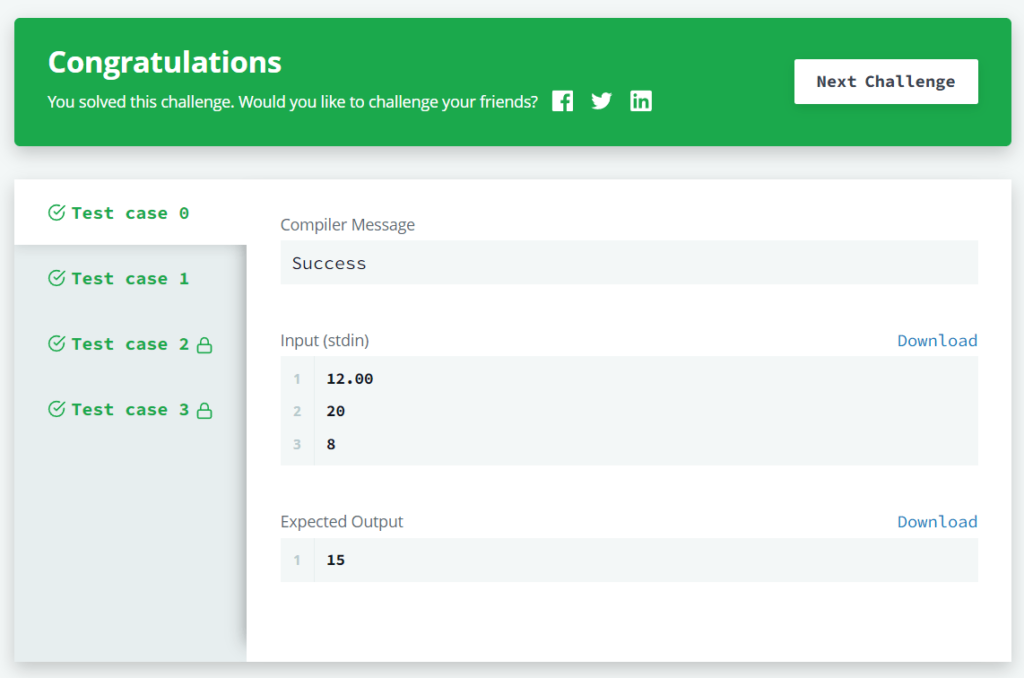
We hope that we bring some value to your life through posting our content, might content meet your expectations. You can always comment on the post to give feedback or reach out to us through email for sharing what you like to read on our blog.
Reach out to us using email: [email protected]
Find More Articles on Our Website: EGrasps
Check out our other posts on Medium.
You can reach out to us on WhatsApp.