In this article, we will be solving HackerRank Day 3: Intro To Conditional Statements problem, part of 30 Days of Code. Majorly, we will use Python3 and Java15 to solve the problem.
Objective
In this challenge, we learn about conditional statements. Check out the Tutorial tab for learning materials and an instructional video.
Task:
Given an integer, n, perform the following conditional actions:
- If n is odd, print
Weird
- If n is even and in the inclusive range of 2 to 5, print
Not Weird
- If n is even and in the inclusive range of 6 to 20, print
Weird
- If n is even and greater than 20, print
Not Weird
Complete the stub code provided in your editor to print whether or not n is weird.
Input Format
A single line containing a positive integer, n.
Constraints
- 1 <= n <= 100
Output Format
Print Weird if the number is weird; otherwise print Not Weird.
Sample Input 0
0
Sample Output 0
Weird
Sample Input 1
24
Sample Output 1
Not Weird
Explanation
Sample Case 0: n = 3
n is odd and odd numbers are weird, so we print Weird
.
Sample Case 1: n = 24
n > 20 and n is even, so it is not weird. This, we print Not Weird
.
You can find all the source code on my GitHub profile: https://github.com/uttammanani/HackerRank-30-Days-of-Code
Solution Day 3: Intro To Conditional Statements in Python3
#!/bin/python3
import math
import os
import random
import re
import sys
if __name__ == '__main__':
N = int(input().strip())
if(N%2 == 1):
print("Weird")
elif(N%2==0) and (N in range(2,7)):
print("Not Weird")
elif(N%2==0) and (N in range(6,21)):
print("Weird")
elif(N%2==0) and (N>20):
print("Not Weird")
Solution Day 3: Intro To Conditional Statements in Java15
import java.io.*;
import java.math.*;
import java.security.*;
import java.text.*;
import java.util.*;
import java.util.concurrent.*;
import java.util.function.*;
import java.util.regex.*;
import java.util.stream.*;
import static java.util.stream.Collectors.joining;
import static java.util.stream.Collectors.toList;
public class Day3 {
public static void main(String[] args) throws IOException {
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(System.in));
int N = Integer.parseInt(bufferedReader.readLine().trim());
Scanner scan = new Scanner(System.in);
if (N % 2 == 0) {
if (N < 6) System.out.println("Not Weird");
else if (N < 21) System.out.println("Weird");
else System.out.println("Not Weird");
} else System.out.println("Weird");
bufferedReader.close();
scan.close();
}
}
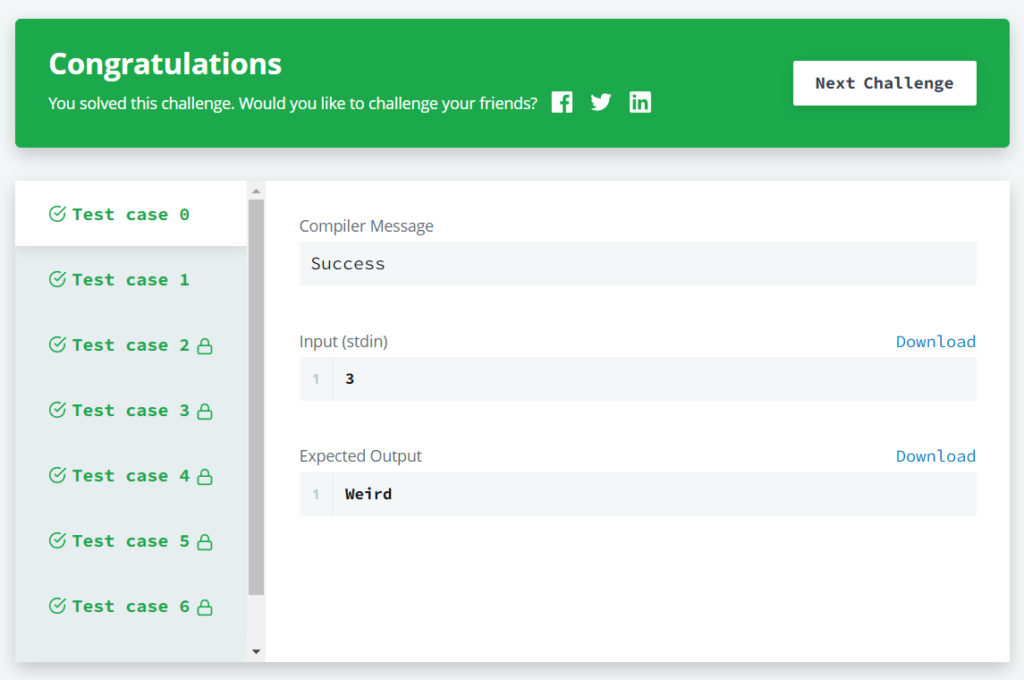
We hope that we bring some value to your life through posting our content, might content meet your expectations. You can always comment on the post to give feedback or reach out to us through email for sharing what you like to read on our blog.
Reach out to us using email: [email protected]
Find More Articles on Our Website: EGrasps
Check out our other posts on Medium.
You can reach out to us on WhatsApp.