In this article, we will be solving HackerRank Day 10: Binary Numbers problem, part of 30 Days of Code. Majorly, we will use Python3 and Java15 to solve the problem.
Objective:
Today, we’re working with binary numbers. Check out the Tutorial tab for learning materials and an instructional video!
Task
Given a base-10 integer, n, convert it to binary (base-2). Then find and print the base-10 integer denoting the maximum number of consecutive 1‘s in n‘s binary representation. When working with different bases, it is common to show the base as a subscript.
Example
n = 125
The binary representation of 12510 is 11111012. In base 10, there are 5 and 1 consecutive ones on two groups. Print the maximum, 5.
Input Format
A single integer, n.
Constraints
- 1 <= n <= 106
Output Format
Print a single base-10 integer that denotes the maximum number of consecutive 1’s in the binary representation of n.
Sample Input 1
5
Sample Output 1
1
Sample Input 2
13
Sample Output 2
2
Explanation
Sample Case 1:
The binary representation of 510 is 1012, so the maximum number of consecutive 1’s is 1.
Sample Case 2:
The binary representation of 1310 is 11012, so the maximum number of consecutive 1’s is 2.
You can find all the source code on my GitHub profile: https://github.com/uttammanani/HackerRank-30-Days-of-Code
Solution Day 10: Binary Numbers in Python3
#!/bin/python3
import math
import os
import random
import re
import sys
if __name__ == '__main__':
n = int(input().strip())
numbers = str(bin(n)[2:]).split('0')
lenghts = [len(num) for num in numbers]
print(max(lenghts))
Solution Day 10: Binary Numbers in Java15
import java.io.*;
import java.math.*;
import java.security.*;
import java.text.*;
import java.util.*;
import java.util.concurrent.*;
import java.util.function.*;
import java.util.regex.*;
import java.util.stream.*;
import static java.util.stream.Collectors.joining;
import static java.util.stream.Collectors.toList;
public class Solution {
public static void main(String[] args) throws IOException {
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(System.in));
int n = Integer.parseInt(bufferedReader.readLine().trim());
char[] binary = Integer.toBinaryString(n).toCharArray();
int tmpCount = 0;
int maxCount = 0;
for(int i = 0; i < binary.length; i++){
tmpCount = (binary[i] == '0') ? 0 : tmpCount + 1;
if(tmpCount > maxCount){
maxCount = tmpCount;
}
}
System.out.println(maxCount);
bufferedReader.close();
}
}
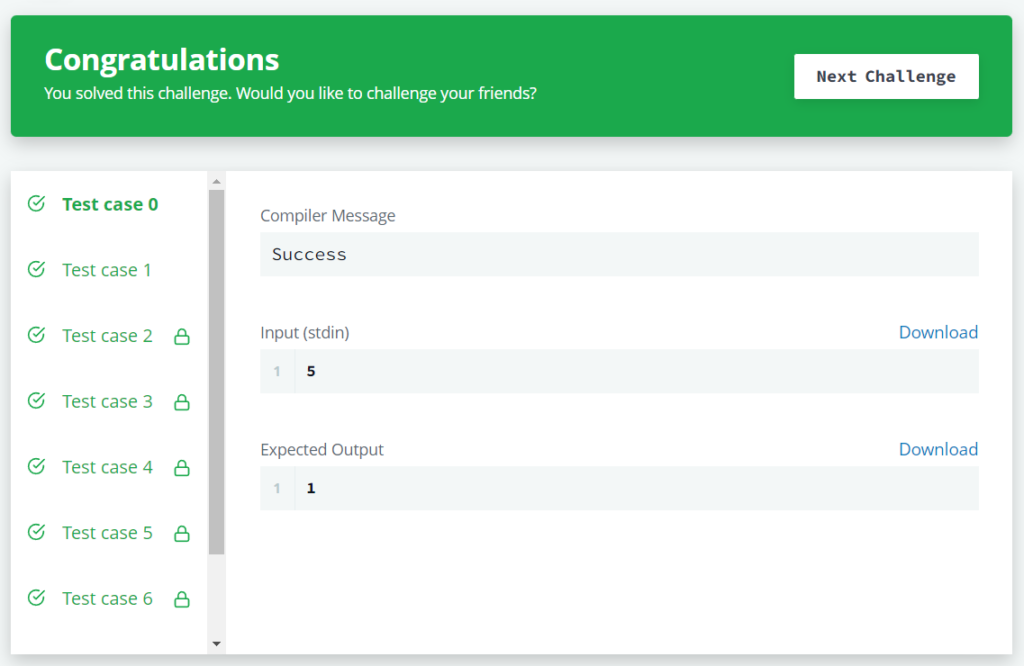
We hope that we bring some value to your life by posting our content, which might meet your expectations. You can always comment on the post to give feedback or reach out to us through email for sharing what you like to read on our blog.
Reach out to us using email: [email protected]
Find More Articles on Our Website: EGrasps
Check out our other posts on Medium.
You can reach out to us on WhatsApp.