In this article, we will be solving HackerRank Day 5: Loops problem, part of 30 Days of Code. Majorly, we will use Python3 and Java15 to solve the problem.
Objective:
In this challenge, we will use loops to do some math. Check out the Tutorial tab to learn more.
Task:
Given an integer, n, print its first 10 multiples. Each multiple n x I (where 1 <= i <= 10) should be printed on a new line in the form: n x i = result
.
Example
n = 3
The printout should look like this:
3 x 1 = 3
3 x 2 = 6
3 x 3 = 9
3 x 4 = 12
3 x 5 = 15
3 x 6 = 18
3 x 7 = 21
3 x 8 = 24
3 x 9 = 27
3 x 10 = 30
Input Format
A single integer, n.
Constraints
- 2 <= n <= 20
Output Format
Print 10 lines of output; each line i (where 1 <= i <= 10) contains the result of n x i in the form: n x i = result
Sample Input
2
Sample Output
2 x 1 = 2
2 x 2 = 4
2 x 3 = 6
2 x 4 = 8
2 x 5 = 10
2 x 6 = 12
2 x 7 = 14
2 x 8 = 16
2 x 9 = 18
2 x 10 = 20
You can find all the source code on my GitHub profile: https://github.com/uttammanani/HackerRank-30-Days-of-Code
Solution Day 5: Loops in Python3
#!/bin/python3
import math
import os
import random
import re
import sys
if __name__ == '__main__':
n = int(input().strip())
i = 1
while (i <= 10):
print(n, "x", i, "=", n*i)
i += 1
Solution Day 5: Loops in Java15
import java.io.*;
import java.math.*;
import java.security.*;
import java.text.*;
import java.util.*;
import java.util.concurrent.*;
import java.util.function.*;
import java.util.regex.*;
import java.util.stream.*;
import static java.util.stream.Collectors.joining;
import static java.util.stream.Collectors.toList;
public class Solution {
public static void main(String[] args) throws IOException {
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(System.in));
int n = Integer.parseInt(bufferedReader.readLine().trim());
for (int i=1; i<=10; i++){
System.out.println(n+ " x " +i+" = " +n*i);
}
bufferedReader.close();
}
}
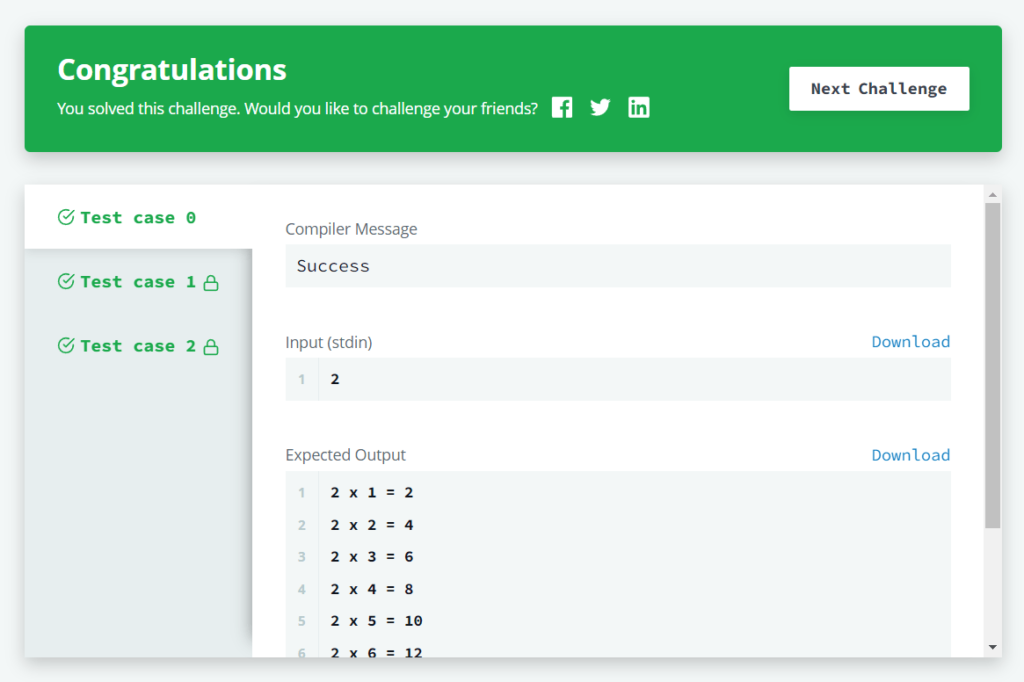
We hope that we bring some value to your life through posting our content, might content meet your expectations. You can always comment on the post to give feedback or reach out to us through email for sharing what you like to read on our blog.
Reach out to us using email: [email protected]
Find More Articles on Our Website: EGrasps
Check out our other posts on Medium.
You can reach out to us on WhatsApp.