In this part of the series Learning Flask, get to learn about how to create and run your very first Flask web application.
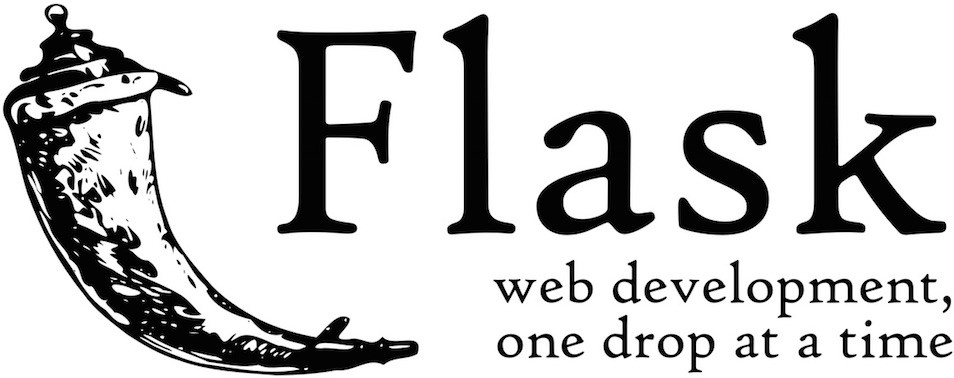
This article will cover how to set up a virtual environment, flask setup, directory, and other basics.
Check Python Installation
Before proceeding with the article make sure you have python installed.
Use this command to check the Python version
python --version
or one can use this command too
python -V
If you do not have python installed, Don’t worry – Read the article on Learn How to install Python on Windows 10/8
Welcome to the Learn Flask series, In this article, we will be creating a very basic web application.
First, we will set up a virtual environment and use it in this series of articles.
One who doesn’t know much don’t worry, we will cover it later.
So Let’s get started.
Creating a Project Directory and Setup Virtual Environment
First, go to the directory where you want to place your project. (Like Downloads, Documents, Desktop, Home Directory)
After that, we need to create the project directory. I am going to name the project directory flask_app.
Note: It would be better if you use the same project name as ours, so, it will be convenient to follow along the way.
Open Terminal/CMD and create the directory with the following command
First change directory in terminal to where you want to save the project and then
mkdir flask_app
Now, Let’s move into the directory we just created
cd flask_app
Let’s create our Python virtual environment with the name flask_venv
python -m venv flask_venv
This command will create a new virtual environment with the name flask_venv
After doing so, You will see a new directory named flask_venv inside our project directory flask_app
For installing flask into our virtual environment we need to activate the virtual environment we just created.
To activate the virtual environment (flask_venv) use the following command from the project directory
Command for Linux and Mac users
source flask_venv/bin/activate (for Linux and Mac user)
Command for Windows users
flask_venv\Scripts\activate (for Windows user)
After that, you can see (flask_venv)
appear in front of your terminal/cmd indicating the virtual environment is activated successfully!
Updating pip
It is best to update pip (which helps in installing the Python’s packages) after creating and activating the new virtual environment (flask_venv). Use command
pip install --upgrade pip
If encounter some errors, You can try this code
python -m pip install --upgrade pip
After successful installation one can see the log like this
Collecting pip
Downloading https://files.pythonhosted.org/packages/47/ca/f0d790b6e18b3a6f3bd5e80c2ee4edbb5807286c21cdd0862ca933f751dd/pip-21.1.3-py3-none-any.whl (1.5MB)
100% |████████████████████████████████| 1.6MB 599kB/s
Installing collected packages: pip
Found existing installation: pip 19.0.3
Uninstalling pip-19.0.3:
Successfully uninstalled pip-19.0.3
Successfully installed pip-21.1.3
Now we can install flask, Let’s install Flask
Installation of Flask in Virtual environment
To install the flask in the virtual environment (flask_venv) use this command
pip install flask
After successful installation of the flask, we can check what libraries (python packages) are installed and it’s the version by using the command
pip list
One can also check the list of installed libraries by using the command
pip freeze
When you use this command you will see plenty of a list of libraries that are installed in the virtual environment
Don’t get confused if you see any new packages as while installing Flask, it also installs few other packages.
Now we have installed the flask, It’s time to create our first flask app.
Create our First Flask app
In this section, we will write a minimalistic app to show Hello World on the web page using Flask.
We will cover in detail and how to do proper structuring in the later part.
So, Let’s get started and create our first app. Make sure to be in the flask_app directory.
One can use the command line (cmd)/Terminal or Text editors like Sublime Text or Visual Studio Code for creating the files and writing the code.
Now create an app.py file, In Linux/Mac systems use this command
touch app.py
Let’s have some fun, now we write some code, open the app.py
we just created in any text editor you prefer.
At first, we are going to import Flask
from flask
from flask import Flask
Now for creating our flask app, we need to pass the __name__ argument to the Flask object and assign it to the variable app.
Do not worry about Why to do this, we will cover this in a later part step by step.
from flask import Flask
app = Flask(__name__)
The next step is to create a route/view for our web application
Let’s do this
from flask import Flask
app = Flask(__name__)
@app.route('/')
def index():
return "Hello World! This is our first Flask Web App"
Understand the code components
Let’s understand the code we have done one by one
@app.route('/')
Routes/View are created using @app.route decorator and passing the endpoint Url or say the path to it in Flask Framework.
In the above example, we have passed the “/” path into the @app.route decorator.
“/” is the root of the flask web app.
This route/view will trigger when someone goes to the index page or root of the web app. (Like in Egrasps we go to https://egrasps.in)
def index():
return "Hello World! This is our first Flask Web App"
Under the @app.route decorator, we have to attach some function that will do something for that route.
So we have written a python function with a return statement.
Flask will return the values which are passed in the return statement, In our case “Hello World! This is our first Flask Web App” string.
Now let’s add more code that enables our app to kickstart and see in the web browser
if __name__ == "__main__":
app.run()
Here __name__ is the special variable used by the python interpreter to provide the functionality of the main function.
For more information on __name__, one can refer to this article: __name__ (A Special variable) in Python
Full Code for First Web app using Flask
After adding all the code, The full file should be looks like
from flask import Flask
app = Flask(__name__)
@app.route("/")
def index():
return "Hello World! This is our first Flask Web App"
if __name__ == "__main__":
app.run()
Voila! We have now successfully written the code for our first web app.
Now, It’s time for running the app.
Running the Flask App
We can run our app in various ways. Let’s check it out.
In the terminal, just check once you’re in the same directory app.py
If you are in the same directory as app.py then run the command:
python app.py
After running this command, If all the setups are as per instruction then you should see something similar to this
* Serving Flask app 'app' (lazy loading)
* Environment: production
WARNING: This is a development server. Do not use it in a production deployment.
Use a production WSGI server instead.
* Debug mode: off
* Running on http://127.0.0.1:5000/ (Press CTRL+C to quit)
If you see so, Congratulations. You have just completed this and successfully build the web app.
Check the Web App in Browser
Now, Let’s check it out in a web browser like chrome browser
Open the chrome browser or any other browser like New Edge or Firefox and paste the URL
In the Browser, you should see the text:
Hello World! This is our first Flask Web App
After this go back to the terminal to stop the app use button from the keyboard “Control + c”
We have seen the one way to run our first flask app, but it is not recommended.
it’s time to learn another way (A better way) which should be used.
Setting Flask environment variables
We set a couple of environment variables in our shell. Which makes running our app even easier.
Run the following commands for setting it up
export FLASK_APP=app.py
export FLASK_ENV=development
The first command will set the app.py value for the FLASK_APP variable.
Use second command will set the development value for FLASK_ENV.
So, basically, we are telling flask that we want to run our app in development mode.
Note: Do not run a live Flask application in production using development mode. Use a production WSGI server instead.
Now we run our app using the following code (the second way)
flask run
After successfully doing this, You should see something like this
* Serving Flask app 'app.py' (lazy loading)
* Environment: development
* Debug mode: on
* Running on http://127.0.0.1:5000/ (Press CTRL+C to quit)
* Restarting with stat
* Debugger is active!
* Debugger PIN: 572-466-591
Have you noticed? That is now the warning you have seen while running using the first method.
Again you can check by going back to the browser and heading to the Url
In the Browser, you should see the text:
Hello World! This is our first Flask Web App
Now go back to the terminal to stop the app use button from the keyboard “Control + c”
Deactivate Virtual Environment
To deactivate the virtual environment, use the command
deactivate
Wrap Up
Now you have seen how to create and run a basic Flask Web Application.
What we have covered in this post
- Check Python Installation
- Create Project directory
- Setting up virtual environment
- Update the PIP library
- Installation of Flask framework (library)
- Write code for first Flask web app
- Understand each component of the code for Flask web app
- Run the Flask App
- Check web app in Browser
- Set Flask environment variable
- Deactivate environment variable
We hope that we bring some value to your life through posting our content, might content meet your expectations. You can always comment on the post to give feedback or reach out to us through email for sharing what you like to read on our blog.
Reach out to us using email: [email protected]
Find More Articles on Our Website: EGrasps
Check out our other posts on Medium.
You can reach out to us on WhatsApp.